Building a Text-Based Chess AI Using AI and Machine Learning
In this tutorial, we’ll guide you through the process of creating your very own computer chess player using the power of Artificial Intelligence (AI) and Machine Learning (ML). Chess is a game that requires strategy and critical thinking, making it a perfect playground for AI. By the end of this tutorial, you’ll have a basic understanding of how to get started with your own text-based chess AI.
Prerequisites:
Before we dive in, let’s ensure you have a good foundation:
- Basic understanding of programming concepts (in Python).
- Comfortable with using the command-line interface (CLI) for running scripts.
Data Collection
To begin, let’s collect a dataset of chess games. You can find a treasure trove of historical games from online sources, chess databases, or even APIs that provide recent games.
Data Preprocessing
Once you have your dataset, we need to make it machine-readable. We’ll use a common format called Portable Game Notation (PGN). PGN represents chess games as a sequence of moves and outcomes. Extract key information such as moves, results (win/lose/draw), and player ratings.
Feature Engineering
The AI needs to understand the game board. Create a representation of the board state and available moves. This is crucial for your AI to make informed decisions.
Model Selection
Now comes the exciting part—selecting an AI model. For beginners, Decision Trees or Random Forests are great starting points. These algorithms learn patterns from data and are relatively easy to implement. For more advanced users, Convolutional Neural Networks (CNNs) or Reinforcement Learning (RL) models like AlphaZero are worth exploring.
Training
Train your chosen model with the preprocessed data. During training, the model learns strategies and patterns from historical games. Think of it as teaching your AI the logic behind chess moves.
Evaluation
Assess your AI’s performance. Pit it against other chess engines or even human players. Measure its performance in terms of wins, losses, and draws. This evaluation helps you understand how effective your AI is becoming.
Fine-Tuning
Refine your AI’s performance by adjusting various parameters. Tweak learning rates, tree depth (for decision trees), or network architecture (for neural networks). Observe how these adjustments impact the AI’s playing strength.
Iterative Process
Remember that building a strong AI takes time and iterations. Continuously train, evaluate, and refine your AI model to enhance its capabilities.
User Interface
Create a simple text-based interface for users to interact with your AI. You can utilize Python’s input()
function to receive moves and showcase the AI’s responses.
Deployment
Once you’re satisfied with your AI’s performance, share your creation! Develop a Python script that allows others to play against your AI in the command line.
Ongoing Maintenance
The journey doesn’t end here. Keep learning and updating your AI. Explore advanced techniques, delve into graphical interfaces, and consider contributing to the broader chess AI community.
Here’s an example
Of how you might use a basic Decision Tree model in Python to create a text-based chess AI. This example provides a simplified illustration of the process, and in reality, chess AI development is far more complex.
from sklearn.tree import DecisionTreeClassifier
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# Sample chess data (features: board state, labels: next move)
X = [[0, 1, 0, 0, 0, 0, 0, 0, 0], # Board state for a move
[0, 1, 0, 0, 0, 0, 0, 0, 0],
...
]
y = [5, 10, ...] # Labels: indices representing next moves
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create a Decision Tree model
model = DecisionTreeClassifier()
# Train the model
model.fit(X_train, y_train)
# Predict moves for test data
predictions = model.predict(X_test)
# Calculate accuracy
accuracy = accuracy_score(y_test, predictions)
print(f"Model Accuracy: {accuracy:.2f}")
This example shows a simplified Decision Tree model where the features (X) represent a board state and the labels (y) are indices representing possible next moves. The model is trained on the data and then used to predict moves on the test set.
Remember, creating a functional chess AI involves more complex feature engineering, larger datasets, and advanced techniques. However, this example should give you a basic idea of how Machine Learning can be applied to chess AI development using Python.
By following this guide, you’ve successfully developed a text-based chess AI using the power of AI and ML. You’ve not only learned about programming and AI but also tapped into the thrilling world of AI-powered game playing. As you continue on your AI journey, remember that this is just the beginning, and the possibilities are endless.
Let’s dive deeper into the role of Machine Learning (ML) within the context of creating a text-based chess AI using the guide:
Model Selection
Machine Learning (ML) is the heart of your chess AI. It’s the technology that enables your AI to learn from historical chess games and make informed decisions. ML algorithms learn patterns and strategies from data, which in this case are the moves and outcomes of chess games.
For beginners, two ML algorithms stand out:
- Decision Trees: Think of these as a flowchart of decisions. The AI learns to ask a series of questions to arrive at a move. For instance, “Is the opponent’s queen threatened?” Based on the answers, the AI chooses the best move. Decision Trees are intuitive and relatively easy to grasp.
- Random Forests: This is an ensemble of multiple decision trees. Each tree makes its own prediction, and the final move is determined by the consensus of the trees. Random Forests tend to be more robust and accurate than individual decision trees.
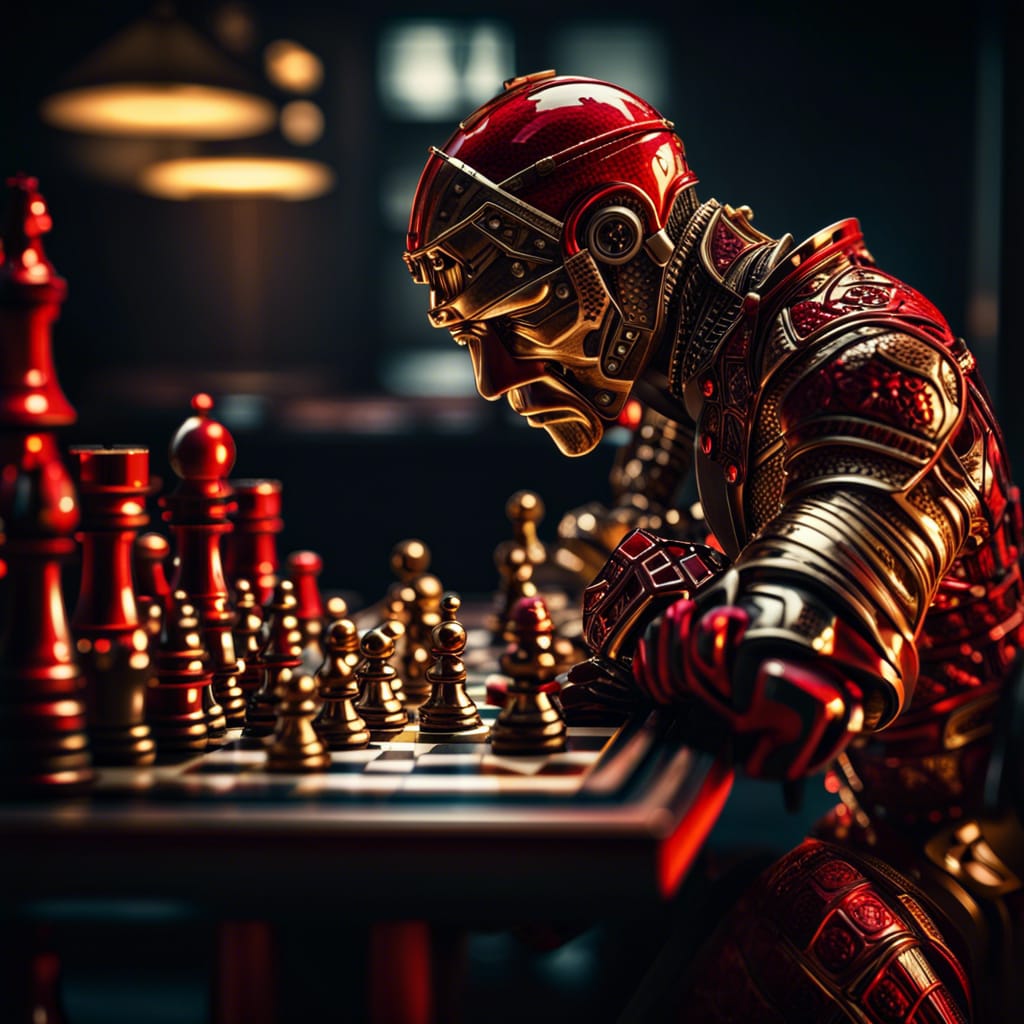
Training
Training is where your AI learns the strategies and patterns from the historical chess games you’ve collected. During this phase, the AI fine-tunes its internal parameters to make better predictions. For instance, it learns that certain moves are often advantageous and others are not.
Evaluation
After training, it’s important to assess how well your AI performs. You can pit it against other chess engines or human players. ML algorithms are designed to generalize from training data to new situations, so evaluation ensures your AI is doing just that—making smart moves beyond what it was specifically trained on.
Fine-Tuning
Fine-tuning involves adjusting parameters that impact how your AI makes decisions. For instance, in Decision Trees, you might control the depth of the tree, which affects the complexity of the decisions. In Neural Networks, you could adjust the learning rate, which determines how quickly the AI adapts to new information.
Iterative Process
Machine Learning is an iterative journey. You train your model, evaluate its performance, and then refine it through fine-tuning. As you iterate, your AI becomes better at understanding chess patterns and making strategic decisions.
Ongoing Maintenance
The field of Machine Learning is always evolving. As you continue your AI journey, you might explore more advanced ML techniques like Neural Networks. Staying updated with the latest research and techniques will help you improve your AI’s performance over time.
Machine Learning empowers your text-based chess AI to learn from data and improve its decision-making abilities. It’s the engine that takes historical games and transforms them into a capable opponent. As you progress, you’ll discover that the nuances of ML add depth and complexity to your AI’s gameplay.
Leave a Reply